UITableView and UITableViewCell Customization in Swift
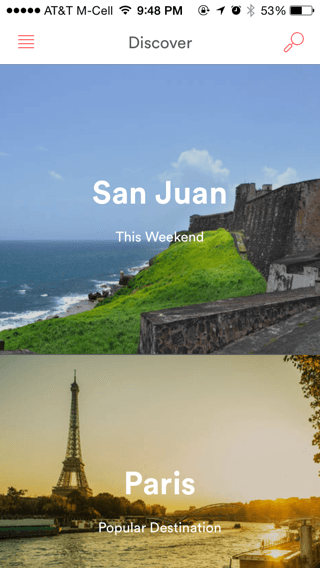
<Image alt="UITableView and UITableViewCell Customization in Swift" objectFit="contain" src="/static/images/airbnb.png" height={350} width={1000} placeholder="blur" quality={100} />
Building Airbnb Discover Page
Our version
<Image alt="UITableView and UITableViewCell Customization in Swift" objectFit="contain" src="/static/images/ut1.png" height={350} width={1000} placeholder="blur" quality={100} />
We will be using the UITableView and custom UITableViewCell to implement this page. One way to make your app standout from the crowd is by uitableviewcell customization, and many feature likes autolayouts play and important role to make it as easy as possible. Lets get started.
- Create a single view application
- Delete the existing view controller on the main storyboard
- Drag a table view controller on to the main storyboard and make it the initial view controller
- Select the UITableViewCell and change the height of the cell to 300
<Image alt="UITableView and UITableViewCell Customization in Swift" objectFit="contain" src="/static/images/ut2.png" height={350} width={1000} placeholder="blur" quality={100} />
- Drag imageview on the UITableViewCell . Select the pin icon and set the make sure the Trailing,Leading, Top and Bottom space to 0. Also unclick the constraints to margin.
<Image alt="UITableView and UITableViewCell Customization in Swift" objectFit="contain" src="/static/images/ut3.png" height={350} width={1000} placeholder="blur" quality={100} />
- Drag Label on the UITableViewCell.
<Image alt="UITableView and UITableViewCell Customization in Swift" objectFit="contain" src="/static/images/ut4.png" height={350} width={1000} placeholder="blur" quality={100} />
- Create a model for the list of places
- Create a new class subclassing UITableViewController and call it PlacesTableViewController
- Create a new class subclassing UITableViewCell and call it PlacesTableViewCell
- Select the UITableViewController and assign the class PlacesTableViewController in identity inspector
- Select the Table view cell and assign the class PlacesTableViewCell in identity inspector
- Create
IBOutlet
from imageview and label to the PlacesTableViewCell. - Select the tableviewcontroller > Go to Editor > Embed in Navigation Controller
- Add the Discover title on the navigation bar. Also add two bar button items on the navigation bar for search and bookmark.
In case you are looking for the application of static tableview or grouped tableview. Find out more on how to build a beautiful settings page here Xcode 6 Tutorial : Grouped UITableView
Data.swift
Just make sure you add 4 images to your project and have the name bridge.jpeg
, mountain.jpeg
, snow.jpeg
and sunset.jpeg
. You can go and find images here Stock Images.
import Foundation
class Data {
class Entry {
let filename : String
let heading : String
init(fname : String, heading : String) {
self.heading = heading
self.filename = fname
}
}
let places = \[
Entry(fname: "bridge.jpeg", heading: "Heading 1"),
Entry(fname: "mountain.jpeg", heading: "Heading 2"),
Entry(fname: "snow.jpeg", heading: "Heading 3"),
Entry(fname: "sunset.jpeg", heading: "Heading 4")
\]
}
PlacesTableViewCell.
import UIKit
class PlacesTableViewCell: UITableViewCell {
@IBOutlet weak var bkImageView: UIImageView!
@IBOutlet weak var headingLabel: UILabel!
override func awakeFromNib() {
super.awakeFromNib()
}
override func setSelected(selected: Bool, animated: Bool) {
super.setSelected(selected, animated: animated)
}
}
PlacesTableViewController
By default tableview cell are seperated by a line. We could remove that line by setting seperatorStyle
to UITableViewCellSeparatorStyle.None
import UIKit
class PlacesTableViewController: UITableViewController {
let data = Data()
override func viewDidLoad() {
super.viewDidLoad()
self.tableView.separatorStyle = UITableViewCellSeparatorStyle.None
}
override func numberOfSectionsInTableView(tableView: UITableView) -> Int {
return 1
}
override func tableView(tableView: UITableView, numberOfRowsInSection section: Int) -> Int {
return data.places.count
}
override func tableView(tableView: UITableView, cellForRowAtIndexPath indexPath: NSIndexPath) -> UITableViewCell {
let cell = tableView.dequeueReusableCellWithIdentifier("Cell", forIndexPath: indexPath) as PlacesTableViewCell
let entry = data.places\[indexPath.row\]
let image = UIImage(named: entry.filename)
cell.bkImageView.image = image
cell.headingLabel.text = entry.heading
return cell
}
}