Swift Tutorial : CoreLocation and Region Monitoring in iOS 8
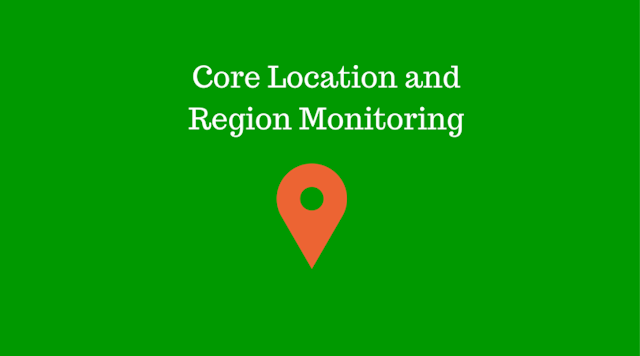
<Image alt="Swift Tutorial : CoreLocation and Region Monitoring in iOS 8" objectFit="contain" src="/static/images/corelocation.png" height={350} width={1000} placeholder="blur" quality={100} />
CoreLocation and Region Monitoring
We will learn how to use CoreLocation and Region Monitoring in Swift. You will be able to change your app to accommodate breaking changes in Core Location.
Location Authorization
Request for authorization for all location functionality. The authorization message can be customized. In iOS 8 there are two Authorization types
- When in use
- Always Authorization
Prerequisites for getting CoreLocation to work in iOS 8
Info.plist keys
Two news keys are added to the info.plist and one of them is mandatory if you want to use location in your app.
NSLocationAlwaysUsageDescription
NSLocationWhenInUseUsageDescription
The value of the keys is the message which you want to display when requesting the authorization.
<Image alt="Swift Tutorial : CoreLocation and Region Monitoring in iOS 8" objectFit="contain" src="/static/images/cl1.png" height={350} width={1000} placeholder="blur" quality={100} />
Add Require Frameworks
Add CoreLocation and MapKit(If you want to display a map) to the Build Phases
<Image alt="Swift Tutorial : CoreLocation and Region Monitoring in iOS 8" objectFit="contain" src="/static/images/cl2.png" height={350} width={1000} placeholder="blur" quality={100} />
Enable Background mode
If you want any features for signification location changes or region monitoring make sure you enable the background mode to location in the capabilities tab.
<Image alt="Swift Tutorial : CoreLocation and Region Monitoring in iOS 8" objectFit="contain" src="/static/images/cl3.png" height={350} width={1000} placeholder="blur" quality={100} />
Creating a GPX file.
Xcode allows you to add custom gpx file to simulate location. To create a gpx file go to http://gpx-poi.com/ provide the longitude and latitude and any other features, then select for mac and download the xml file. And then add it to Xcode as described below.
You can edit the scheme to make this newly added gpx location as your default location.
<Image alt="Swift Tutorial : CoreLocation and Region Monitoring in iOS 8" objectFit="contain" src="/static/images/cl4.png" height={350} width={1000} placeholder="blur" quality={100} />
Lets look at flow for getting any location information
<iframe src="http://www.youtube.com/embed/brp3h7iR5Kg" width="320" height="215" frameborder="0" allowfullscreen="allowfullscreen" ></iframe>If you are interested in learning iOS Development I suggest you take a look at [Building an app every month in 2015]
For CoreLocation
- Make sure the view controller conforms to protocol CLLocationManagerDelegate.
- Set the CLLocationManager delegate to self.
- Set the desired accuracy to best
- If you app will access the location only in the foreground or when the app is in use, request for
requestWhenInUseAuthorization()
. - Finally you need to implement
func locationManager(manager: CLLocationManager!, didUpdateLocations locations: [AnyObject]!)
- If you want to display the map view we could use the location coordinate which we just got in
didUpdateLocation
and set it up as a mapView region as shown in the code below.
For Region Monitoring
- Make sure the view controller conforms to protocol CLLocationManagerDelegate.
- Set the CLLocationManager delegate to self.
- Set the desired accuracy to best
- We need to request for
requestAlwaysAuthorization()
. - Finally you need to implement didEnterRegion and didExitRegion and put any custom login in those functions.
func locationManager(manager: CLLocationManager!, didEnterRegion region: CLRegion!) {
NSLog("Entering region")
}
func locationManager(manager: CLLocationManager!, didExitRegion region: CLRegion!) {
NSLog("Exit region")
}
I add my home location as the default location and when I enable the region monitoring I could change the location in the xcode to simulate entering and leaving my home.
//
// ViewController.swift
// CoreLocation
//
// Created by Shrikar Archak on 12/27/14.
// Copyright (c) 2014 Shrikar Archak. All rights reserved.
//
import UIKit
import CoreLocation
import MapKit
class ViewController: UIViewController, CLLocationManagerDelegate {
var manager: CLLocationManager?
@IBOutlet weak var mapView: MKMapView!
@IBOutlet weak var address: UILabel!
override func viewDidLoad() {
super.viewDidLoad()
manager = CLLocationManager()
manager?.delegate = self;
manager?.desiredAccuracy = kCLLocationAccuracyBest
}
override func didReceiveMemoryWarning() {
super.didReceiveMemoryWarning()
// Dispose of any resources that can be recreated.
}
@IBAction func getLocation(sender: AnyObject) {
let available = CLLocationManager.isMonitoringAvailableForClass(CLCircularRegion)
manager?.requestWhenInUseAuthorization()
manager?.startUpdatingLocation()
}
@IBAction func regionMonitoring(sender: AnyObject) {
manager?.requestAlwaysAuthorization()
let currRegion = CLCircularRegion(center: CLLocationCoordinate2D(latitude: 37.411822, longitude: -121.941125), radius: 200, identifier: "Home")
manager?.startMonitoringForRegion(currRegion)
}
func locationManager(manager: CLLocationManager!, didUpdateLocations locations: \[AnyObject\]!) {
manager.stopUpdatingLocation()
let location = locations\[0\] as CLLocation
let geoCoder = CLGeocoder()
geoCoder.reverseGeocodeLocation(location, completionHandler: { (data, error) -> Void in
let placeMarks = data as \[CLPlacemark\]
let loc: CLPlacemark = placeMarks\[0\]
self.mapView.centerCoordinate = location.coordinate
let addr = loc.locality
self.address.text = addr
let reg = MKCoordinateRegionMakeWithDistance(location.coordinate, 1500, 1500)
self.mapView.setRegion(reg, animated: true)
self.mapView.showsUserLocation = true
})
}
func locationManager(manager: CLLocationManager!, didEnterRegion region: CLRegion!) {
NSLog("Entering region")
}
func locationManager(manager: CLLocationManager!, didExitRegion region: CLRegion!) {
NSLog("Exit region")
}
func locationManager(manager: CLLocationManager!, didFailWithError error: NSError!) {
NSLog("\\(error)")
}
}
Please let me know if you have any questions/feedback.