iOS Development Tutorial : UIActionSheet and UIAlertController in Swift
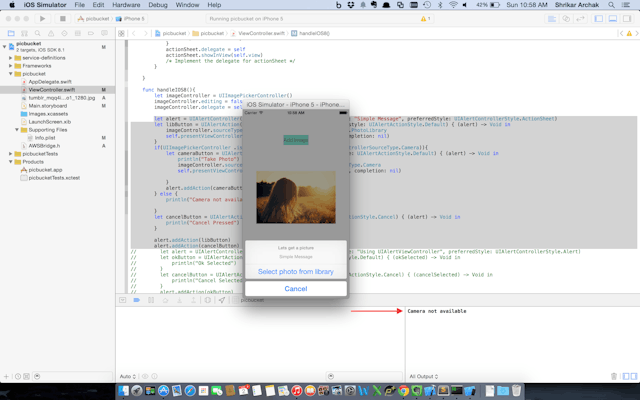
<Image alt="UIActionSheet and UIAlertController in Swift" objectFit="cover" src="/static/images/UIpic4.png" height={250} width={1000} placeholder="blur" quality={100} />
In this iOS Development Tutorial series we will learn how to use UIActionSheet and UIAlertController in Swift.
What we will cover
- Creating App
- UIAlertView using UIAlertController
- UIActionSheet using UIAlertController
- Using UIAlertView/UIActionSheet without breaking the code for iOS 7
UIAlertView and UIActionSheet are deprecated in iOS 8 and are replaced by UIAlertController.
We use UIAlertView/UIActionSheet/UIAlertController to allow user to perform interactions in your app.
UIAlertView is used to alert user about something that happened within your app, the operations you can do when displaying an alertview is very limited. Normally you tend to have an acknowledge button like OK and more more button to CANCEL or dismiss the current interaction.
UIActionSheet is another way you can provide interactions to the user in you app. Example being selecting a photo to upload. You can allow interactions like “Select Picture from the photo library” or provide the user to take a picture.
Create App
Let go ahead and create a new project picbucket.
<Image alt="UIActionSheet and UIAlertController in Swift" objectFit="cover" src="/static/images/UIpic1.png" height={250} width={1000} placeholder="blur" quality={100} />
UIAlertView using UIAlertController
A UIAlertController object displays an alert message to the user. This class replaces the UIActionSheet and UIAlertView classes for displaying alerts. After configuring the alert controller with the actions and style you want, present it using the presentViewController:animated:completion: method.
title: The title of the alert to get user’s attention message: Detailed message for the alert style : can be UIAlertControllerStyle.Alert
If you are interested in learning iOS Development I suggest you take a look at [Building an app every month in 2015]
We use completion handler to perform actions depending on what the user selected.
let alert = UIAlertController(title: "This is an alert!", message: "Using UIAlertController", preferredStyle: UIAlertControllerStyle.Alert)
let okButton = UIAlertAction(title: "OK", style: UIAlertActionStyle.Default) { (okSelected) -> Void in
println("Ok Selected")
}
let cancelButton = UIAlertAction(title: "Cancel", style: UIAlertActionStyle.Cancel) { (cancelSelected) -> Void in
println("Cancel Selected")
}
alert.addAction(okButton)
alert.addAction(cancelButton)
self.presentViewController(alert, animated: true, completion: nil)
<Image alt="UIActionSheet and UIAlertController in Swift" objectFit="cover" src="/static/images/UIpic3.png" height={250} width={1000} placeholder="blur" quality={100} />
UIActionSheet using UIAlertController
Similary we can create an UIActionSheet using the code below title: The title of the alert to get user’s attention message: Detailed message for the alert style : UIAlertControllerStyle.ActionSheet
let alert = UIAlertController(title: "Lets get a picture", message: "Simple Message", preferredStyle: UIAlertControllerStyle.ActionSheet)
let libButton = UIAlertAction(title: "Select photo from library", style: UIAlertActionStyle.Default) { (alert) -> Void in
imageController.sourceType = UIImagePickerControllerSourceType.PhotoLibrary
self.presentViewController(imageController, animated: true, completion: nil)
}
if(UIImagePickerController .isSourceTypeAvailable(UIImagePickerControllerSourceType.Camera)){
let cameraButton = UIAlertAction(title: "Take a picture", style: UIAlertActionStyle.Default) { (alert) -> Void in
println("Take Photo")
imageController.sourceType = UIImagePickerControllerSourceType.Camera
self.presentViewController(imageController, animated: true, completion: nil)
}
alert.addAction(cameraButton)
} else {
println("Camera not available")
}
let cancelButton = UIAlertAction(title: "Cancel", style: UIAlertActionStyle.Cancel) { (alert) -> Void in
println("Cancel Pressed")
}
alert.addAction(libButton)
alert.addAction(cancelButton)
self.presentViewController(alert, animated: true, completion: nil)
In the above example we have certain conditional which will help us to add new button conditionally. In our example we will add the “Take a picture button” only if camera is available. This code works properly and add “Take a picture” button on the device and you guys just need to trust me on that.
<Image alt="UIActionSheet and UIAlertController in Swift" objectFit="cover" src="/static/images/UIpic4.png" height={250} width={1000} placeholder="blur" quality={100} />
How to provide backward compatibility in iOS 7
Since UIAlertController is not available in iOS7 and the above code will crash if you run it on iOS7. Let see how to use UIAlertView and UIActionSheet across iOS7 and iOS8 effectively. controllerAvailable is a helper function which will help us branch our code depending on the available feature for that iOS.
func controllerAvailable() -> Bool {
if let gotModernAlert: AnyClass = NSClassFromString("UIAlertController") {
return true;
}
else {
return false;
}
}
For iOS 7 we need to implement the UIActionSheetDelegate and the func actionSheet(actionSheet: UIActionSheet, clickedButtonAtIndex buttonIndex: Int)
.
//
// ViewController.swift
// picbucket
//
// Created by Shrikar Archak on 11/1/14.
// Copyright (c) 2014 Shrikar Archak. All rights reserved.
//
import UIKit
class ViewController: UIViewController, UINavigationControllerDelegate, UIImagePickerControllerDelegate, UIActionSheetDelegate {
@IBOutlet weak var selectedImage: UIImageView!
@IBAction func selectImage(sender: AnyObject) {
/\* Supports UIAlert Controller \*/
if( controllerAvailable()){
handleIOS8()
} else {
var actionSheet:UIActionSheet
if(UIImagePickerController .isSourceTypeAvailable(UIImagePickerControllerSourceType.Camera)){
actionSheet = UIActionSheet(title: "Hello this is IOS7", delegate: self, cancelButtonTitle: "Cancel", destructiveButtonTitle: nil,otherButtonTitles:"Select photo from library", "Take a picture")
} else {
actionSheet = UIActionSheet(title: "Hello this is IOS7", delegate: self, cancelButtonTitle: "Cancel", destructiveButtonTitle: nil,otherButtonTitles:"Select photo from library")
}
actionSheet.delegate = self
actionSheet.showInView(self.view)
/\* Implement the delegate for actionSheet \*/
}
}
func handleIOS8(){
let imageController = UIImagePickerController()
imageController.editing = false
imageController.delegate = self;
let alert = UIAlertController(title: "Lets get a picture", message: "Simple Message", preferredStyle: UIAlertControllerStyle.ActionSheet)
let libButton = UIAlertAction(title: "Select photo from library", style: UIAlertActionStyle.Default) { (alert) -> Void in
imageController.sourceType = UIImagePickerControllerSourceType.PhotoLibrary
self.presentViewController(imageController, animated: true, completion: nil)
}
if(UIImagePickerController .isSourceTypeAvailable(UIImagePickerControllerSourceType.Camera)){
let cameraButton = UIAlertAction(title: "Take a picture", style: UIAlertActionStyle.Default) { (alert) -> Void in
println("Take Photo")
imageController.sourceType = UIImagePickerControllerSourceType.Camera
self.presentViewController(imageController, animated: true, completion: nil)
}
alert.addAction(cameraButton)
} else {
println("Camera not available")
}
let cancelButton = UIAlertAction(title: "Cancel", style: UIAlertActionStyle.Cancel) { (alert) -> Void in
println("Cancel Pressed")
}
alert.addAction(libButton)
alert.addAction(cancelButton)
/\* Code for UIAlert View Controller
let alert = UIAlertController(title: "This is an alert!", message: "Using UIAlertController", preferredStyle: UIAlertControllerStyle.Alert)
let okButton = UIAlertAction(title: "OK", style: UIAlertActionStyle.Default) { (okSelected) -> Void in
println("Ok Selected")
}
let cancelButton = UIAlertAction(title: "Cancel", style: UIAlertActionStyle.Cancel) { (cancelSelected) -> Void in
println("Cancel Selected")
}
alert.addAction(okButton)
alert.addAction(cancelButton)
\*/
self.presentViewController(alert, animated: true, completion: nil)
}
func imagePickerController(picker: UIImagePickerController!, didFinishPickingImage image: UIImage!, editingInfo: \[NSObject : AnyObject\]!) {
self.dismissViewControllerAnimated(true, nil)
self.selectedImage.image = image;
}
func actionSheet(actionSheet: UIActionSheet, clickedButtonAtIndex buttonIndex: Int) {
println("Title : \\(actionSheet.buttonTitleAtIndex(buttonIndex))")
println("Button Index : \\(buttonIndex)")
let imageController = UIImagePickerController()
imageController.editing = false
imageController.delegate = self;
if( buttonIndex == 1){
imageController.sourceType = UIImagePickerControllerSourceType.PhotoLibrary
} else if(buttonIndex == 2){
imageController.sourceType = UIImagePickerControllerSourceType.Camera
} else {
}
self.presentViewController(imageController, animated: true, completion: nil)
}
func controllerAvailable() -> Bool {
if let gotModernAlert: AnyClass = NSClassFromString("UIAlertController") {
return true;
}
else {
return false;
}
}
override func viewDidLoad() {
super.viewDidLoad()
}
override func didReceiveMemoryWarning() {
super.didReceiveMemoryWarning()
// Dispose of any resources that can be recreated.
}
}
For Complete code take a look here <Link href="https://github.com/sarchak/picbucket" color="blue.500" fontWeight="bold"> Github Repo</Link>. Also do comment if I am missing something or for any feedback.
<iframe src="//www.youtube.com/embed/O4QVAmDYvkU" width="420" height="315" frameborder="0" ></iframe>