iOS 8 Notifications in Swift
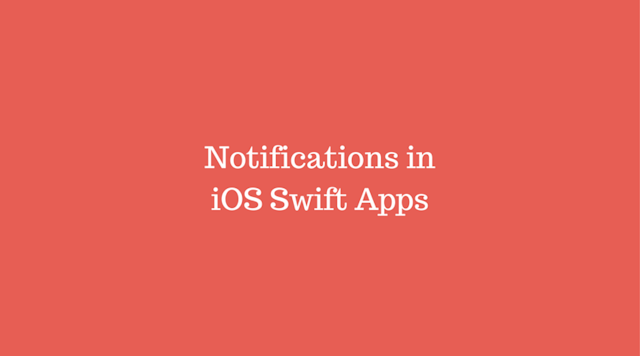
iOS 8 Notifications in Swift
In this iOS Development tutorial series we will look at notifications and how to use them in our Swift app.
User Notification
These are the interactions which user can interact with or see that are delivered by your app when the app is in the background mode. * Badge Notification * Sound Notification * Alerts
* Modal Alert stay until user interact
* Alert in the notification center
* Alert displayed on the lock screen.
User notification can be delivered locally using UILocalNotification or remotely using Push Notification
Registering for User Notification
func application(application: UIApplication, didFinishLaunchingWithOptions launchOptions: [NSObject: AnyObject]?) -> Bool { let notificationType = UIUserNotificationType.Alert | UIUserNotificationType.Badge | UIUserNotificationType.Sound let settings = UIUserNotificationSettings(forTypes: notificationType, categories: nil) application.registerUserNotificationSettings(settings) return true }
After the alert for getting the permission is shown the didRegisterUserNotificationSettings
is called with the necessary notification settings the user has allowed.
Notification Actions
In this section we will describe the workflow for dealing with Notification Actions.
- Register for Notification Action
- Push/Schedule Notification
- Handle Notification Action
If you are interested in learning iOS Development I suggest you take a look at [Building an app every month in 2015]
Register for Notification Actions
let notificationType = UIUserNotificationType.Alert | UIUserNotificationType.Badge | UIUserNotificationType.Sound let acceptAction = UIMutableUserNotificationAction() acceptAction.identifier = "Accept" acceptAction.title = "Accept" acceptAction.activationMode = UIUserNotificationActivationMode.Background acceptAction.destructive = false acceptAction.authenticationRequired = false
let declineAction = UIMutableUserNotificationAction() declineAction.identifier = "Decline" declineAction.title = "Decline" declineAction.activationMode = UIUserNotificationActivationMode.Background declineAction.destructive = false declineAction.authenticationRequired = false
let category = UIMutableUserNotificationCategory() category.identifier = "invite" category.setActions([acceptAction, declineAction], forContext: UIUserNotificationActionContext.Default) let categories = NSSet(array: [category]) let settings = UIUserNotificationSettings(forTypes: notificationType, categories: categories) application.registerUserNotificationSettings(settings)
Schedule Local Notification
The next step once we have configured the settings is to schedule local notification. Make sure the category is what we have configured in our case invite
identifier.
var localNotification:UILocalNotification = UILocalNotification() localNotification.alertAction = "Testing notifications on iOS8" localNotification.alertBody = "Local notifications are working" localNotification.fireDate = NSDate(timeIntervalSinceNow: 5) localNotification.soundName = UILocalNotificationDefaultSoundName localNotification.category = "invite" UIApplication.sharedApplication().scheduleLocalNotification(localNotification)
Handle Local Notification
After we schedule the notification we need to perform certain action depending on what option the user selected. We need to implement the function handleActionWithIdentifier
which gets called when the user selects any of the option.
func application(application: UIApplication, handleActionWithIdentifier identifier: String?, forLocalNotification notification: UILocalNotification, completionHandler: () -> Void) { NSLog("Handle identifier : \(identifier)") // Must be called when finished completionHandler() }
Remote Notification
This by itself needs a complete blog post take a look at Remote Notification or Tuts+ Remote Notification
Location Notification
Location notification helps app in triggering a notification when the user enter a particular region. Location notification can be configured to trigger once or multiple times.
User should register for Core Location Authorization before the Location notification can be used.
Prerequisite
Registering core location.
let locationManager = CLLocationManager() locationManager.delegate = self locationManager.requestWhenInUseAuthorization()
Scheduling Local Notification
One thing to note is that when scheduling a location notification make sure you dont have a firedate set for the local notification as it would crash if we set both the fire data as well as the region together.
var localNotification:UILocalNotification = UILocalNotification() localNotification.regionTriggersOnce = true localNotification.region = CLCircularRegion(circularRegionWithCenter: CLLocationCoordinate2D(latitude: 37.33233141, longitude: -122.03121860), radius: 50.0, identifier: "Location1") UIApplication.sharedApplication().scheduleLocalNotification(localNotification)
Let me know if you have any questions / suggestion in the comments below.
[mailmunch-form id="67365"]